Introduction :
Encryption of information on web is very crucial part nowdays , laravel comes with Crypt facade to implement encryption in laravel.
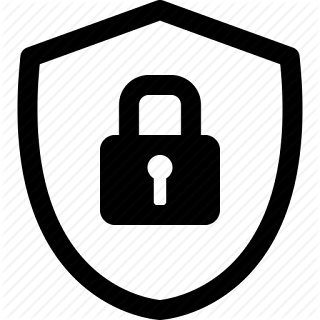
Usage:
- Step 1 : First set key in config\app.php with random string . key should be 32 characters
- Step 2 : Use Crypt facade for encryption and decryption.
Encryption :
$encrypted_value=Crypt::decrypt($request->password);
Decryption :
$decrypted_value=Crypt::decrypt($encrypted_value);
Example :
namespace App\Http\Controllers;
use Crypt;
use App\User;
use Illuminate\Http\Request;
use App\Http\Controllers\Controller;
use Illuminate\Contracts\Encryption\DecryptException;
class UserController extends Controller
{
public function storeSecret(Request $request, $id)
{
$user = User::findOrFail($id);
$encryptedValue=Crypt::encrypt($request->secret);
$user->fill([
'secret' =>$encryptedValue
])->save();
echo ‘Encrypted value : ’.$encryptedValue.’
’;
try {
$decrypted = Crypt::decrypt();
echo ‘decrypted value : ’.$decrypted.’
’;
} catch (DecryptException $e) {
}
}
}